create a file name upload.php in the same folder where the uploads folder is created.
Place the code in the file named upload.php
$target_path = "uploads/";
$target_path = $target_path . basename( $_FILES['uploadedfile']['name']);
if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $target_path)) {
echo "The file ". basename( $_FILES['uploadedfile']['name']).
" has been uploaded";
} else{
echo "There was an error uploading the file, please try again!";
}
Client side code that is used to send file to a server. please include the following code in our client program.
private void doFileUpload(){
HttpURLConnection conn = null;
DataOutputStream dos = null;
DataInputStream inStream = null;
// The full path of filename which is to be uploaded to server
String exsistingFileName = "test.mp3";
String lineEnd = "\r\n";
String twoHyphens = "--";
String boundary = "*****";
int bytesRead, bytesAvailable, bufferSize;
byte[] buffer;
int maxBufferSize = 1*1024*1024;
String responseFromServer = "";
//Server path to the upload.php
String urlString = "http://xxx.xxx.xxx.xxx/upload.php";
try
{
//------------------ CLIENT REQUEST
FileInputStream fileInputStream = new FileInputStream(new File(exsistingFileName) );
// open a URL connection to the Servlet
URL url = new URL(urlString);
// Open a HTTP connection to the URL
conn = (HttpURLConnection) url.openConnection();
// Allow Inputs
conn.setDoInput(true);
// Allow Outputs
conn.setDoOutput(true);
// Don't use a cached copy.
conn.setUseCaches(false);
// Use a post method.
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Content-Type", "multipart/form-data;boundary="+boundary);
dos = new DataOutputStream( conn.getOutputStream() );
dos.writeBytes(twoHyphens + boundary + lineEnd);
dos.writeBytes("Content-Disposition: form-data; name=\"uploadedfile\";filename=\"" + exsistingFileName +"\"" + lineEnd);
dos.writeBytes(lineEnd);
System.out.println("Headers are written");
// create a buffer of maximum size
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
buffer = new byte[bufferSize];
// read file and write it into form...
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
while (bytesRead > 0)
{
dos.write(buffer, 0, bufferSize);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable, maxBufferSize);
bytesRead = fileInputStream.read(buffer, 0, bufferSize);
}
// send multipart form data necesssary after file data...
dos.writeBytes(lineEnd);
dos.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd);
// close streams
System.out.println("File is written");
fileInputStream.close();
dos.flush();
dos.close();
}
catch (MalformedURLException ex)
{
System.out.println(ex.toString());
}
catch (IOException ioe)
{
System.out.println(ioe.toString());
}
//------------------ read the SERVER RESPONSE
try {
inStream = new DataInputStream ( conn.getInputStream() );
String str;
while (( str = inStream.readLine()) != null)
{
//the str contains the server response
System.out.println("Server Response"+str);
}
inStream.close();
}
catch (IOException ioex){
System.out.println(ioe.toString());
}
}
If the file is sucessfully uploaded the response will be
The file test.mp3 has been uploaded.
otherwise the response will be
There was an error uploading the file, please try again!
Original Message
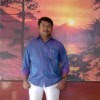
hai i need a help from u and u are the only person to give correct solution to me thanks
ReplyDeletei use your code to uploads a file to social web sites its a whootin web
i get error
and they give a url its decription to me its below
POST files/new
Uploads new file to user's Files section. Your POST request's Content-Type should be set to multipart/form-data with the file parameter.
Resource URL
http://whootin.com/api/v1/files/new.format (json | xml)
Parameters
file
required A file to upload.
folder_id
optional A folder to upload to.
Example Request
POST http:/whootin.com/api/v1/files/new.json
POST Data